Note
Go to the end to download the full example code
09: Reporting & Referencing¶
This section covers how to access reporting info and reference use of the module.
This page is a hands-on example of the reporting and referencing information on the Reference page.
# Import FOOOF model objects
from fooof import FOOOF, FOOOFGroup
# Import simulation functions to create some example data
from fooof.sim import gen_power_spectrum, gen_group_power_spectra
# Import utilities to print out information for reporting
from fooof.utils.reports import methods_report_info, methods_report_text
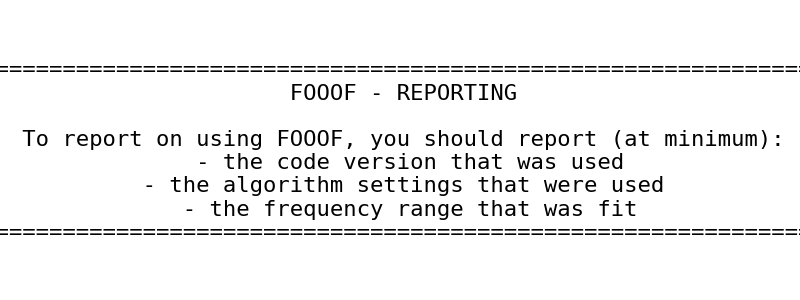
Checking Module Version¶
It’s useful and important to keep track of which version of the module you are using, and to report this information when referencing use of the tool.
There are several ways to check the version of the the module that you are using, including checking what is installed in the Python environment you are using.
From within Python, you can also check the version of the module by checking __version__, as shown below:
# Check the version of the module
from fooof import __version__ as fooof_version
print('Current fooof version:', fooof_version)
Current fooof version: 1.1.0
Getting Model Reporting Information¶
To assist with reporting information, the module has the following utilities:
the
methods_report_info()
, which prints out methods reporting informationthe
methods_report_text()
, which prints out a template of methods reporting text
Both of the these functions take as input a model object, and use the object to collect and return information related to the model fits.
Note that not all information may be returned by the model - these methods only have access to the current object. This means it is also important that if you use these functions, you use them with objects that match the settings and data used in the analysis to be reported.
In the following, we will explore an example of using these functions for an example model fit.
# Print out all the methods information for reporting
methods_report_info(fooof_obj)
==================================================================================================
FOOOF - REPORTING
To report on using FOOOF, you should report (at minimum):
- the code version that was used
- the algorithm settings that were used
- the frequency range that was fit
==================================================================================================
==================================================================================================
FOOOF - VERSION
1.1.0
==================================================================================================
==================================================================================================
FOOOF - SETTINGS
Peak Width Limits : (0.5, 12.0)
Max Number of Peaks : inf
Minimum Peak Height : 0.0
Peak Threshold: 2.0
Aperiodic Mode : fixed
==================================================================================================
==================================================================================================
FOOOF - FIT RANGE
The model was fit from XX to XX Hz.
==================================================================================================
You might notice that the above function prints out several different sections, some of which might look familiar.
The settings information, for example, is the same as printed using the
# - print_settings()
method.
Next, let’s check out the text version of the methods report.
# Generate methods text, with methods information inserted
methods_report_text(fooof_obj)
The FOOOF algorithm (version 1.1.0) was used to parameterize neural power spectra. Settings for the algorithm were set as: peak width limits : (0.5, 12.0); max number of peaks : inf; minimum peak height : 0.0; peak threshold : 2.0; and aperiodic mode : 'fixed'. Power spectra were parameterized across the frequency range XX to XX Hz.
Additional Examples¶
In the above examples, not all the information was printed, as not all information was available in the example object (for example, it had no data).
In the next example, let’s see how the outputs look for an example object with model fit results.
# Simulate an example power spectrum
freqs, powers = gen_power_spectrum([1, 50], [0, 10, 1], [10, 0.25, 2], freq_res=0.25)
# Initialize model object
fm = FOOOF(min_peak_height=0.1, peak_width_limits=[1, 6], aperiodic_mode='knee')
fm.fit(freqs, powers)
# Generate methods text, with methods information inserted
methods_report_info(fm)
==================================================================================================
FOOOF - REPORTING
To report on using FOOOF, you should report (at minimum):
- the code version that was used
- the algorithm settings that were used
- the frequency range that was fit
==================================================================================================
==================================================================================================
FOOOF - VERSION
1.1.0
==================================================================================================
==================================================================================================
FOOOF - SETTINGS
Peak Width Limits : [1, 6]
Max Number of Peaks : inf
Minimum Peak Height : 0.1
Peak Threshold: 2.0
Aperiodic Mode : knee
==================================================================================================
==================================================================================================
FOOOF - FIT RANGE
The model was fit from 1.0 to 50.0 Hz.
==================================================================================================
# Generate methods text, with methods information inserted
methods_report_text(fm)
The FOOOF algorithm (version 1.1.0) was used to parameterize neural power spectra. Settings for the algorithm were set as: peak width limits : [1, 6]; max number of peaks : inf; minimum peak height : 0.1; peak threshold : 2.0; and aperiodic mode : 'knee'. Power spectra were parameterized across the frequency range 1.0 to 50.0 Hz.
The report text is meant as a template / example that could be added to a methods section.
Note that there may be missing information that needs to be filled in (indicated by ‘XX’s), and you can and should consider this a template to be edited as needed.
Other Model Objects¶
Note that the reporting functions work with any model object.
For example, next we will use them on a FOOOFGroup
object.
# Simulate an example group of power spectra
freqs, powers = gen_group_power_spectra(10, [1, 75], [0, 1], [10, 0.25, 2])
# Initialize and fit group model object
fg = FOOOFGroup(max_n_peaks=4, peak_threshold=1.75)
fg.fit(freqs, powers)
Running FOOOFGroup across 10 power spectra.
FOOOF WARNING: Lower-bound peak width limit is < or ~= the frequency resolution: 0.50 <= 0.50
Lower bounds below frequency-resolution have no effect (effective lower bound is the frequency resolution).
Too low a limit may lead to overfitting noise as small bandwidth peaks.
We recommend a lower bound of approximately 2x the frequency resolution.
# Generate the methods report information
methods_report_info(fg)
==================================================================================================
FOOOF - REPORTING
To report on using FOOOF, you should report (at minimum):
- the code version that was used
- the algorithm settings that were used
- the frequency range that was fit
==================================================================================================
==================================================================================================
FOOOF - VERSION
1.1.0
==================================================================================================
==================================================================================================
FOOOF - SETTINGS
Peak Width Limits : (0.5, 12.0)
Max Number of Peaks : 4
Minimum Peak Height : 0.0
Peak Threshold: 1.75
Aperiodic Mode : fixed
==================================================================================================
==================================================================================================
FOOOF - FIT RANGE
The model was fit from 1.0 to 75.0 Hz.
==================================================================================================
# Generate the methods report text
methods_report_text(fg)
The FOOOF algorithm (version 1.1.0) was used to parameterize neural power spectra. Settings for the algorithm were set as: peak width limits : (0.5, 12.0); max number of peaks : 4; minimum peak height : 0.0; peak threshold : 1.75; and aperiodic mode : 'fixed'. Power spectra were parameterized across the frequency range 1.0 to 75.0 Hz.
That concludes the example of using the helper utilities for generating methods reports!
Total running time of the script: ( 0 minutes 0.133 seconds)